Zen Editor Update: Context Menu Hell
So I finally shipped a new Zen Editor update that adds a proper context menu to the file tree. Holy fucking shit, this was a nightmare to implement. What should have been a standard feature took me way longer than I expected.
New Context Menu Features
Despite all the pain and suffering, I managed to add a proper context menu to the file tree with these features:
- Open - Opens the selected file in the editor (was already there)
- Copy Path - Now copies the full path instead of just the name
- Reveal in Explorer - Finally works properly to show the file in your system file explorer
- Delete - Removes files with status bar confirmation (press Y/N), not a dialog
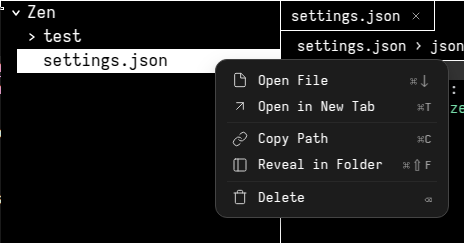
The new context menu in Zen Editor
Why No Rename Feature?
So you're probably wondering, "Why the fuck isn't there a rename feature? That's like the most basic thing ever." Well, let me tell you about the fucking nightmare I've been dealing with...
The Tab System From Hell
The current tab system in Zen Editor is completely broken when it comes to tracking files. When you rename a file, the tab still points to the old filename, and the whole system loses track of the file. I tried for hours to fix this shit but ran into problem after problem:
- File watchers don't get properly updated with the new path
- Path normalization issues between Windows and Unix systems
- Monaco editor models don't update correctly
- Tabs keep their old path references
- Everything explodes if you have multiple tabs open
I sat there from 4 AM to 7 AM trying to solve this bullshit, getting more and more pissed off as each "solution" led to two more problems. Finally, I said "fuck it" and decided to ship what works now and rewrite the entire tab system later.
What Actually Works
Despite the rename feature being a complete disaster, the rest of the context menu actually works pretty well:
Feature | Status | Notes |
---|---|---|
Context Menu UI | ✓ | Opens on right-click, closes properly when clicking elsewhere |
Open Files | ✓ | Already worked before, but now integrated in menu |
Copy Path | ✓ | Now copies full path instead of just filename |
Reveal in Explorer | ✓ | Fixed to work on all platforms correctly |
Delete | ✓ | Uses status bar confirmation (Y/N) instead of dialog |
Rename | ✗ | Completely broken due to tab system issues |

File deletion with status bar confirmation
Properly Closing When Clicking Elsewhere
One of the most annoying problems was that the context menu wouldn't close when you clicked somewhere else. This is now fixed with a proper global click handler:
function showContextMenu(event, items) {
// Prevent default context menu
event.preventDefault();
// Position the context menu at the cursor position
const menu = document.getElementById('context-menu');
menu.style.left = `${event.pageX}px`;
menu.style.top = `${event.pageY}px`;
// Populate the menu with items
populateContextMenu(items);
// Show the menu
menu.style.display = 'block';
// Add a global click handler to close the menu when clicking elsewhere
// Using setTimeout to avoid triggering on the current click event
setTimeout(() => {
window.addEventListener('click', closeContextMenuOnClick);
}, 100);
}
function closeContextMenuOnClick(event) {
const menu = document.getElementById('context-menu');
// Close the menu if the click is outside the menu
if (menu && !menu.contains(event.target)) {
hideContextMenu();
}
}
function hideContextMenu() {
const menu = document.getElementById('context-menu');
if (menu) {
menu.style.display = 'none';
}
// Remove global click handler
window.removeEventListener('click', closeContextMenuOnClick);
}
Next Steps
So what's next? I need to completely rewrite the tab system to properly handle file operations, including:
- Proper path tracking that handles renames
- Better editor model management
- File watcher system that actually works
- Tab state persistence that isn't a complete mess
If you're feeling brave enough to try the current version, it's available on GitHub. Just don't try to rename files or you'll break everything.
Final Thoughts
This update should have been straightforward, but it turned into a complete nightmare. The good news is I've learned a lot about what not to do when designing an editor. Sometimes you have to build something shitty to understand how to make it good.
Until next time, when I'll hopefully have fixed the fucking rename feature.